Steps to set up a new Laravel app, initialize a git repo, push to Github
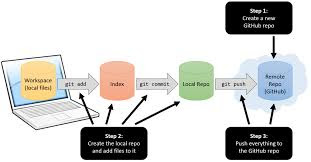
This is a list of steps to: Setup a new Laravel app Initialize a local repository using git Create a new remote repository using GitHub Change README.rdoc Assumptions: PHP is installed Composer is installed Git is installed Github account is established Setup a new Laravel app Navigate to the directory in which you want the new app created using 'change directory' (cd). Use the 'make directory' (mkdir) command if you want to create a new directory, such as laravel_projects (Note: Laravel will automatically create a directory for all your app files) $ cd <correct_directory> Create a new app. It's good practice to append your new app name with 'blog' so that it will not be confused with any classes you create later. Installing Laravel Laravel utilizes Composer to manage its dependencies. So, before using Laravel, make sure you have Composer installed on your machine. Via Laravel Installer ...